Aim :
Write a C/C++ program to set up a real-time clock interval timer using the alarm API.
Theory :
- First, every signal has a name. These names all begin with the three characters SIG .For example,SIGABRT is the abort signal that is generated when a process calls the abort function.
- SIGALRM is the alarm signal that is generated when the timer set by the alarm function goes off.
- Use the alarm API for generating a signal after certain time interval as specified by the user.
Code :
#include<stdio.h>
#include<stdlib.h>
#include<unistd.h>
#include<signal.h>
#define INTERVAL 5
void callme(int sig_no)
{
alarm(INTERVAL);
printf("Hello!!\n");
}
int main()
{
struct sigaction action;
action.sa_handler=(void(*)(int))callme;
sigaction(SIGALRM,&action,0);
alarm(2);
sleep(5);
return 0;
}
Output :
- Open a terminal.
- Change directory to the file location in both the terminals.
- Open a file using command followed by program_name
vi 10_alarm_signal_handler.cpp
and then enter the source code and save it.
- Then compile the program using
g++ 10_alarm_signal_handler.cpp
- If there are no errors after compilation execute the program using
./a.out
Screenshot:-
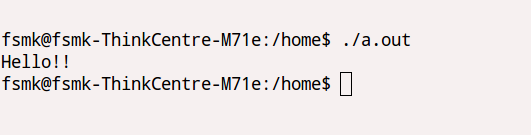